import os
from math import inf
import warnings
"ignore") warnings.filterwarnings(
35 Hyperparameter Tuning with spotpython
and PyTorch
Lightning for the Diabetes Data Set
In this section, we will show how spotpython
can be integrated into the PyTorch
Lightning training workflow for a regression task. It demonstrates how easy it is to use spotpython
to tune hyperparameters for a PyTorch
Lightning model.
35.1 The Basic Setting
After importing the necessary libraries, the fun_control
dictionary is set up via the fun_control_init
function. The fun_control
dictionary contains
PREFIX
: a unique identifier for the experimentfun_evals
: the number of function evaluationsmax_time
: the maximum run time in minutesdata_set
: the data set. Here we use theDiabetes
data set that is provided byspotpython
.core_model_name
: the class name of the neural network model. This neural network model is provided byspotpython
.hyperdict
: the hyperparameter dictionary. This dictionary is used to define the hyperparameters of the neural network model. It is also provided byspotpython
._L_in
: the number of input features. Since theDiabetes
data set has 10 features,_L_in
is set to 10._L_out
: the number of output features. Since we want to predict a single value,_L_out
is set to 1.
The HyperLight
class is used to define the objective function fun
. It connects the PyTorch
and the spotpython
methods and is provided by spotpython
.
from spotpython.data.diabetes import Diabetes
from spotpython.hyperdict.light_hyper_dict import LightHyperDict
from spotpython.fun.hyperlight import HyperLight
from spotpython.utils.init import (fun_control_init, surrogate_control_init, design_control_init)
from spotpython.utils.eda import print_exp_table, print_res_table
from spotpython.spot import Spot
from spotpython.utils.file import get_experiment_filename
="601"
PREFIX
= Diabetes()
data_set
= fun_control_init(
fun_control =PREFIX,
PREFIX=inf,
fun_evals=1,
max_time= data_set,
data_set ="light.regression.NNLinearRegressor",
core_model_name=LightHyperDict,
hyperdict=10,
_L_in=1)
_L_out
= HyperLight().fun fun
module_name: light
submodule_name: regression
model_name: NNLinearRegressor
The method set_hyperparameter
allows the user to modify default hyperparameter settings. Here we modify some hyperparameters to keep the model small and to decrease the tuning time.
from spotpython.hyperparameters.values import set_hyperparameter
"optimizer", [ "Adadelta", "Adam", "Adamax"])
set_hyperparameter(fun_control, "l1", [3,4])
set_hyperparameter(fun_control, "epochs", [3,7])
set_hyperparameter(fun_control, "batch_size", [4,11])
set_hyperparameter(fun_control, "dropout_prob", [0.0, 0.025])
set_hyperparameter(fun_control, "patience", [2,3])
set_hyperparameter(fun_control,
= design_control_init(init_size=10)
design_control print_exp_table(fun_control)
| name | type | default | lower | upper | transform |
|----------------|--------|-----------|---------|---------|-----------------------|
| l1 | int | 3 | 3 | 4 | transform_power_2_int |
| epochs | int | 4 | 3 | 7 | transform_power_2_int |
| batch_size | int | 4 | 4 | 11 | transform_power_2_int |
| act_fn | factor | ReLU | 0 | 5 | None |
| optimizer | factor | SGD | 0 | 2 | None |
| dropout_prob | float | 0.01 | 0 | 0.025 | None |
| lr_mult | float | 1.0 | 0.1 | 10 | None |
| patience | int | 2 | 2 | 3 | transform_power_2_int |
| batch_norm | factor | 0 | 0 | 1 | None |
| initialization | factor | Default | 0 | 4 | None |
Finally, a Spot
object is created. Calling the method run()
starts the hyperparameter tuning process.
= Spot(fun=fun,fun_control=fun_control, design_control=design_control)
S S.run()
train_model result: {'val_loss': 23075.09765625, 'hp_metric': 23075.09765625}
train_model result: {'val_loss': 3466.6259765625, 'hp_metric': 3466.6259765625}
train_model result: {'val_loss': 4775.9482421875, 'hp_metric': 4775.9482421875}
train_model result: {'val_loss': 23974.962890625, 'hp_metric': 23974.962890625}
train_model result: {'val_loss': 22921.740234375, 'hp_metric': 22921.740234375}
train_model result: {'val_loss': 4060.3369140625, 'hp_metric': 4060.3369140625}
train_model result: {'val_loss': 20739.09375, 'hp_metric': 20739.09375}
train_model result: {'val_loss': 4064.956298828125, 'hp_metric': 4064.956298828125}
train_model result: {'val_loss': 20911.953125, 'hp_metric': 20911.953125}
train_model result: {'val_loss': 23951.484375, 'hp_metric': 23951.484375}
train_model result: {'val_loss': 3396.2470703125, 'hp_metric': 3396.2470703125}
spotpython tuning: 3396.2470703125 [----------] 1.94%
train_model result: {'val_loss': nan, 'hp_metric': nan}
train_model result: {'val_loss': nan, 'hp_metric': nan}
spotpython tuning: 3396.2470703125 [----------] 3.40%
train_model result: {'val_loss': 3414.830078125, 'hp_metric': 3414.830078125}
spotpython tuning: 3396.2470703125 [#---------] 6.42%
train_model result: {'val_loss': 23692.912109375, 'hp_metric': 23692.912109375}
spotpython tuning: 3396.2470703125 [#---------] 7.93%
train_model result: {'val_loss': 8255.0693359375, 'hp_metric': 8255.0693359375}
spotpython tuning: 3396.2470703125 [#---------] 10.97%
train_model result: {'val_loss': 17554.509765625, 'hp_metric': 17554.509765625}
spotpython tuning: 3396.2470703125 [#---------] 12.71%
train_model result: {'val_loss': 8407.7724609375, 'hp_metric': 8407.7724609375}
spotpython tuning: 3396.2470703125 [##--------] 15.77%
train_model result: {'val_loss': 2980.89794921875, 'hp_metric': 2980.89794921875}
spotpython tuning: 2980.89794921875 [##--------] 19.39%
train_model result: {'val_loss': 3568.241943359375, 'hp_metric': 3568.241943359375}
spotpython tuning: 2980.89794921875 [##--------] 21.90%
train_model result: {'val_loss': 3090.523193359375, 'hp_metric': 3090.523193359375}
spotpython tuning: 2980.89794921875 [##--------] 24.50%
train_model result: {'val_loss': 12307.81640625, 'hp_metric': 12307.81640625}
spotpython tuning: 2980.89794921875 [###-------] 26.69%
train_model result: {'val_loss': 4920.1708984375, 'hp_metric': 4920.1708984375}
spotpython tuning: 2980.89794921875 [###-------] 29.95%
train_model result: {'val_loss': 8808.490234375, 'hp_metric': 8808.490234375}
spotpython tuning: 2980.89794921875 [####------] 40.65%
train_model result: {'val_loss': 22241.390625, 'hp_metric': 22241.390625}
spotpython tuning: 2980.89794921875 [####------] 43.66%
train_model result: {'val_loss': 3245.012939453125, 'hp_metric': 3245.012939453125}
spotpython tuning: 2980.89794921875 [#####-----] 46.55%
train_model result: {'val_loss': 3300.112548828125, 'hp_metric': 3300.112548828125}
spotpython tuning: 2980.89794921875 [#####-----] 49.83%
train_model result: {'val_loss': 3785.927978515625, 'hp_metric': 3785.927978515625}
spotpython tuning: 2980.89794921875 [#####-----] 53.35%
train_model result: {'val_loss': 1.284459928928387e+18, 'hp_metric': 1.284459928928387e+18}
spotpython tuning: 2980.89794921875 [######----] 56.37%
train_model result: {'val_loss': 5260.1796875, 'hp_metric': 5260.1796875}
spotpython tuning: 2980.89794921875 [######----] 58.50%
train_model result: {'val_loss': 23540.33984375, 'hp_metric': 23540.33984375}
spotpython tuning: 2980.89794921875 [######----] 61.84%
train_model result: {'val_loss': 6140.5615234375, 'hp_metric': 6140.5615234375}
spotpython tuning: 2980.89794921875 [#######---] 68.53%
train_model result: {'val_loss': 16852.33984375, 'hp_metric': 16852.33984375}
spotpython tuning: 2980.89794921875 [#######---] 74.52%
train_model result: {'val_loss': 19499.26171875, 'hp_metric': 19499.26171875}
spotpython tuning: 2980.89794921875 [########--] 80.82%
train_model result: {'val_loss': 3554.0283203125, 'hp_metric': 3554.0283203125}
spotpython tuning: 2980.89794921875 [#########-] 86.03%
train_model result: {'val_loss': 3105.140869140625, 'hp_metric': 3105.140869140625}
spotpython tuning: 2980.89794921875 [#########-] 90.19%
train_model result: {'val_loss': 23906.033203125, 'hp_metric': 23906.033203125}
spotpython tuning: 2980.89794921875 [#########-] 93.75%
train_model result: {'val_loss': 23956.08984375, 'hp_metric': 23956.08984375}
spotpython tuning: 2980.89794921875 [##########] 97.94%
train_model result: {'val_loss': 22778.099609375, 'hp_metric': 22778.099609375}
spotpython tuning: 2980.89794921875 [##########] 100.00% Done...
Experiment saved to 601_res.pkl
<spotpython.spot.spot.Spot at 0x10428e300>
35.2 Looking at the Results
35.2.1 Tuning Progress
After the hyperparameter tuning run is finished, the progress of the hyperparameter tuning can be visualized with spotpython
’s method plot_progress
. The black points represent the performace values (score or metric) of hyperparameter configurations from the initial design, whereas the red points represents the hyperparameter configurations found by the surrogate model based optimization.
S.plot_progress()
35.2.2 Tuned Hyperparameters and Their Importance
Results can be printed in tabular form.
print_res_table(S)
| name | type | default | lower | upper | tuned | transform | importance | stars |
|----------------|--------|-----------|---------|---------|-------------------|-----------------------|--------------|---------|
| l1 | int | 3 | 3.0 | 4.0 | 4.0 | transform_power_2_int | 0.00 | |
| epochs | int | 4 | 3.0 | 7.0 | 4.0 | transform_power_2_int | 0.00 | |
| batch_size | int | 4 | 4.0 | 11.0 | 6.0 | transform_power_2_int | 0.00 | |
| act_fn | factor | ReLU | 0.0 | 5.0 | ReLU | None | 0.00 | |
| optimizer | factor | SGD | 0.0 | 2.0 | Adamax | None | 100.00 | *** |
| dropout_prob | float | 0.01 | 0.0 | 0.025 | 0.025 | None | 0.00 | |
| lr_mult | float | 1.0 | 0.1 | 10.0 | 4.935071237769679 | None | 0.00 | |
| patience | int | 2 | 2.0 | 3.0 | 3.0 | transform_power_2_int | 0.00 | |
| batch_norm | factor | 0 | 0.0 | 1.0 | 0 | None | 71.47 | ** |
| initialization | factor | Default | 0.0 | 4.0 | xavier_normal | None | 100.00 | *** |
A histogram can be used to visualize the most important hyperparameters.
=1.0) S.plot_importance(threshold
=3) S.plot_important_hyperparameter_contour(max_imp
l1: 0.001
epochs: 0.001
batch_size: 0.001
act_fn: 0.001
optimizer: 100.0
dropout_prob: 0.001
lr_mult: 0.001
patience: 0.001
batch_norm: 71.46576951548953
initialization: 100.0
35.2.3 Get the Tuned Architecture
import pprint
from spotpython.hyperparameters.values import get_tuned_architecture
= get_tuned_architecture(S)
config pprint.pprint(config)
{'act_fn': ReLU(),
'batch_norm': False,
'batch_size': 64,
'dropout_prob': 0.025,
'epochs': 16,
'initialization': 'xavier_normal',
'l1': 16,
'lr_mult': 4.935071237769679,
'optimizer': 'Adamax',
'patience': 8}
35.2.4 Test on the full data set
# set the value of the key "TENSORBOARD_CLEAN" to True in the fun_control dictionary and use the update() method to update the fun_control dictionary
"TENSORBOARD_CLEAN": True})
fun_control.update({"tensorboard_log": True}) fun_control.update({
from spotpython.light.testmodel import test_model
from spotpython.utils.init import get_feature_names
test_model(config, fun_control) get_feature_names(fun_control)
┏━━━━━━━━━━━━━━━━━━━━━━━━━━━┳━━━━━━━━━━━━━━━━━━━━━━━━━━━┓ ┃ Test metric ┃ DataLoader 0 ┃ ┡━━━━━━━━━━━━━━━━━━━━━━━━━━━╇━━━━━━━━━━━━━━━━━━━━━━━━━━━┩ │ hp_metric │ 3650.771240234375 │ │ val_loss │ 3650.771240234375 │ └───────────────────────────┴───────────────────────────┘
test_model result: {'val_loss': 3650.771240234375, 'hp_metric': 3650.771240234375}
['age',
'sex',
'bmi',
'bp',
's1_tc',
's2_ldl',
's3_hdl',
's4_tch',
's5_ltg',
's6_glu']
35.3 Cross Validation With Lightning
- The
KFold
class fromsklearn.model_selection
is used to generate the folds for cross-validation. - These mechanism is used to generate the folds for the final evaluation of the model.
- The
CrossValidationDataModule
class [SOURCE] is used to generate the folds for the hyperparameter tuning process. - It is called from the
cv_model
function [SOURCE].
config
{'l1': 16,
'epochs': 16,
'batch_size': 64,
'act_fn': ReLU(),
'optimizer': 'Adamax',
'dropout_prob': 0.025,
'lr_mult': 4.935071237769679,
'patience': 8,
'batch_norm': False,
'initialization': 'xavier_normal'}
from spotpython.light.cvmodel import cv_model
"k_folds": 2})
fun_control.update({"test_size": 0.6})
fun_control.update({ cv_model(config, fun_control)
k: 0
train_model result: {'val_loss': 12037.67578125, 'hp_metric': 12037.67578125}
k: 1
train_model result: {'val_loss': 3403.876953125, 'hp_metric': 3403.876953125}
7720.7763671875
35.4 Extending the Basic Setup
This basic setup can be adapted to user-specific needs in many ways. For example, the user can specify a custom data set, a custom model, or a custom loss function. The following sections provide more details on how to customize the hyperparameter tuning process. Before we proceed, we will provide an overview of the basic settings of the hyperparameter tuning process and explain the parameters used so far.
35.4.1 General Experiment Setup
To keep track of the different experiments, we use a PREFIX
for the experiment name. The PREFIX
is used to create a unique experiment name. The PREFIX
is also used to create a unique TensorBoard folder, which is used to store the TensorBoard log files.
spotpython
allows the specification of two different types of stopping criteria: first, the number of function evaluations (fun_evals
), and second, the maximum run time in seconds (max_time
). Here, we will set the number of function evaluations to infinity and the maximum run time to one minute.
max_time
is set to one minute for demonstration purposes. For real experiments, this value should be increased. Note, the total run time may exceed the specified max_time
, because the initial design is always evaluated, even if this takes longer than max_time
.
35.4.2 Data Setup
Here, we have provided the Diabetes
data set class, which is a subclass of torch.utils.data.Dataset
. Data preprocessing is handled by Lightning
and PyTorch
. It is described in the LIGHTNINGDATAMODULE documentation.
The data splitting, i.e., the generation of training, validation, and testing data, is handled by Lightning
.
35.4.3 Objective Function fun
The objective function fun
from the class HyperLight
[SOURCE] is selected next. It implements an interface from PyTorch
’s training, validation, and testing methods to spotpython
.
35.4.4 Core-Model Setup
By using core_model_name = "light.regression.NNLinearRegressor"
, the spotpython
model class NetLightRegression
[SOURCE] from the light.regression
module is selected.
35.4.5 Hyperdict Setup
For a given core_model_name
, the corresponding hyperparameters are automatically loaded from the associated dictionary, which is stored as a JSON file. The JSON file contains hyperparameter type information, names, and bounds. For spotpython
models, the hyperparameters are stored in the LightHyperDict
, see [SOURCE] Alternatively, you can load a local hyper_dict. The hyperdict
uses the default hyperparameter settings. These can be modified as described in Section D.15.1.
35.4.6 Other Settings
There are several additional parameters that can be specified, e.g., since we did not specify a loss function, mean_squared_error
is used, which is the default loss function. These will be explained in more detail in the following sections.
35.5 Tensorboard
The textual output shown in the console (or code cell) can be visualized with Tensorboard, if the argument tensorboard_log
to fun_control_init()
is set to True
. The Tensorboard log files are stored in the runs
folder. To start Tensorboard, run the following command in the terminal:
tensorboard --logdir="runs/"
Further information can be found in the PyTorch Lightning documentation for Tensorboard.
35.6 Loading the Saved Experiment and Getting the Hyperparameters of the Tuned Model
To get the tuned hyperparameters as a dictionary, the get_tuned_architecture
function can be used.
from spotpython.utils.file import load_result
= load_result(PREFIX=PREFIX)
spot_tuner = get_tuned_architecture(spot_tuner)
config config
Loaded experiment from 601_res.pkl
{'l1': 16,
'epochs': 16,
'batch_size': 64,
'act_fn': ReLU(),
'optimizer': 'Adamax',
'dropout_prob': 0.025,
'lr_mult': 4.935071237769679,
'patience': 8,
'batch_norm': False,
'initialization': 'xavier_normal'}
35.7 Using the spotgui
The spotgui
[github] provides a convenient way to interact with the hyperparameter tuning process. To obtain the settings from Section 35.1, the spotgui
can be started as shown in Figure 35.1.
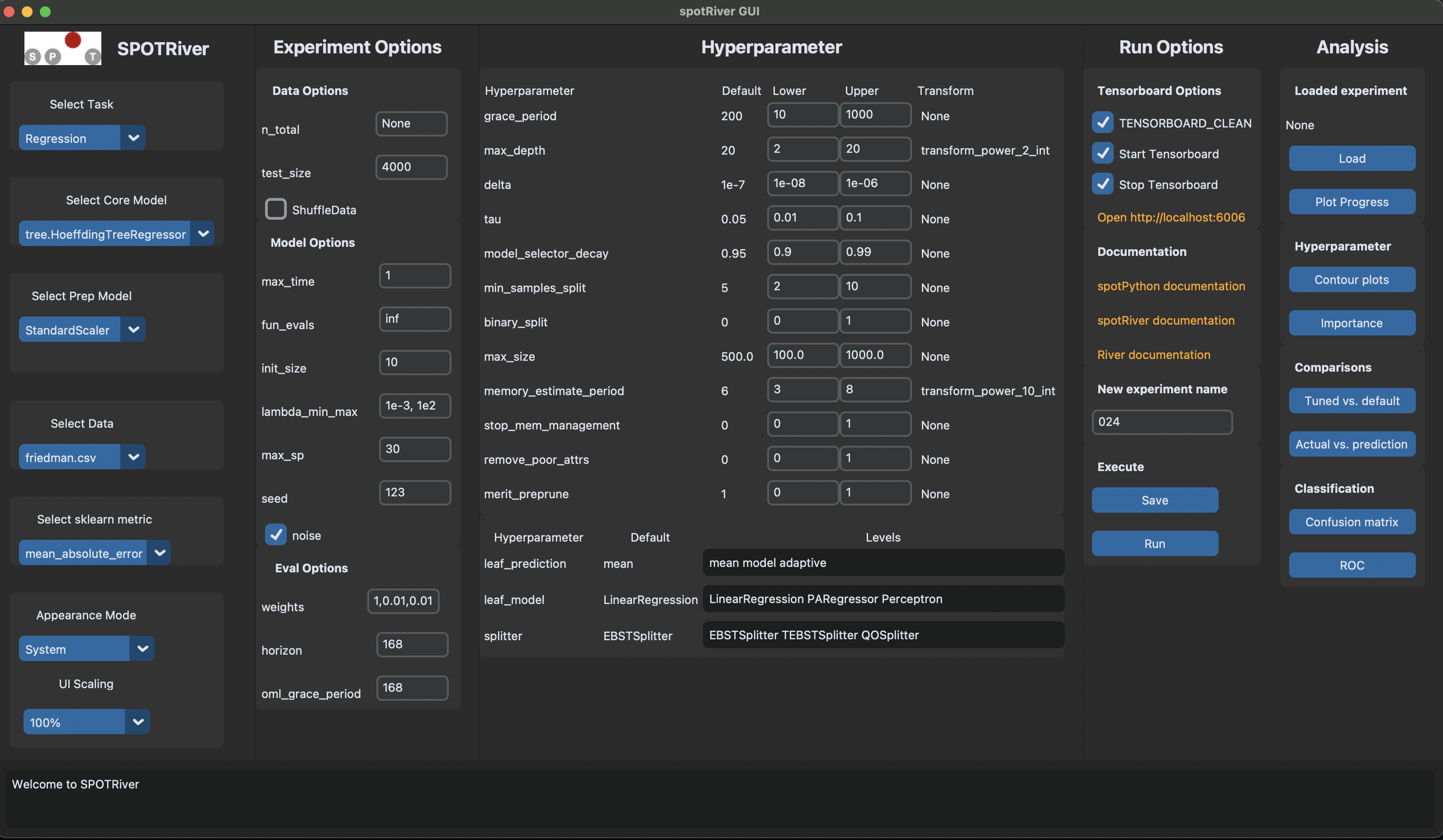
35.8 Summary
This section presented an introduction to the basic setup of hyperparameter tuning with spotpython
and PyTorch
Lightning.